IMPORTANT: This feature works for mpddev versions >= v24.06.24. Do a complete rebuild (rm -rf /mpdroot/build) before using it for the first time.
You have a task you are using in your macro
class MyOwnTask : public FairTask {...}
Your goals are
1. Adding your own histograms, which you can update during each event
2. View those histograms in a .root file after the run finishes
Do:
1. In the constructor of MyOwnTask create your histograms, and add them to fTaskHistograms
MyOwnTask::MyOwnTask(){
TH1F* histo_1d = new TH1F("1D","1D_title",100,-4,4);
fTaskHistograms->Add(histo_1d);
TH2F* histo_2d = new TH2F("2D","2D_title",100,0.,10.,100,0.,10.);
fTaskHistograms->Add(histo_2d);
}
You can then fill and update your histograms anytime during your run.
2. At the end of the run, your histograms are written into file TasksHistograms.root, which you can view in TBrowser.
– each task has its own directory with all of the task histograms
– task directories are ordered and named after MyOwnTask::GetName()
Simple exercise:
Add the code in red into digitizer’s constructor, and check the generated TasksHistograms.root file
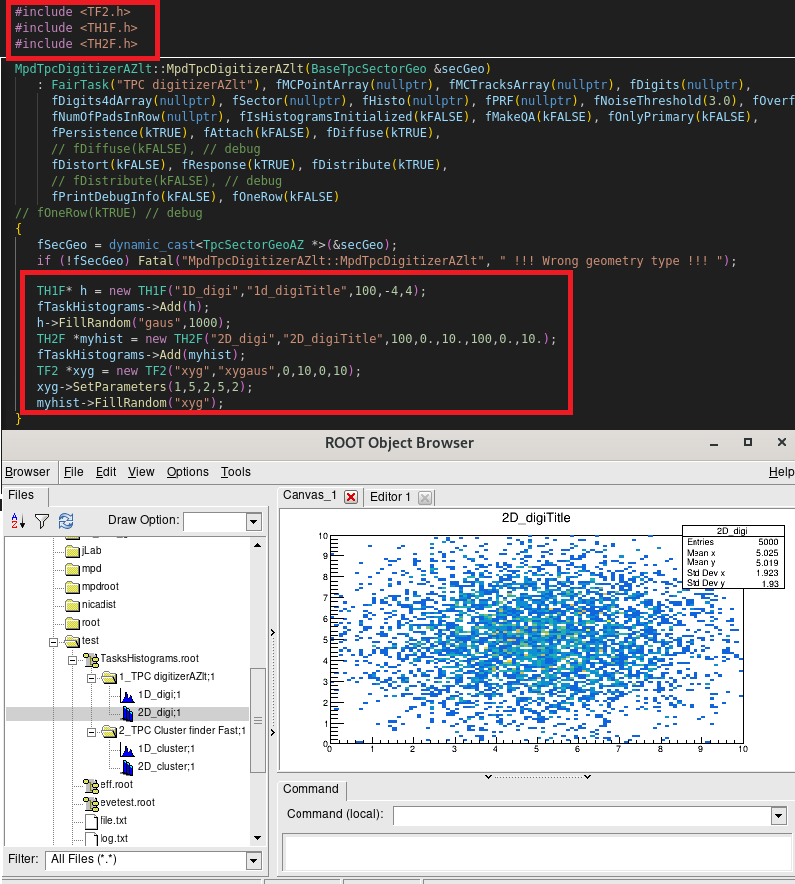
For your convenience, the selected chunks of code for copy/paste-ing:
#include <TH1F.h>
#include <TH2F.h>
#include <TF2.h>
TH1F* h = new TH1F("1D_digi","1d_digiTitle",100,-4,4);
fTaskHistograms->Add(h);
h->FillRandom("gaus",1000);
TH2F *myhist = new TH2F("2D_digi","2D_digiTitle",100,0.,10.,100,0.,10.);
fTaskHistograms->Add(myhist);
TF2 *xyg = new TF2("xyg","xygaus",0,10,0,10);
xyg->SetParameters(1,5,2,5,2);
myhist->FillRandom("xyg");